Step 6
Note the following variables are employed to hold the state of the game:
const numRows = 3;
const numCols = 3;
let numEmptyCells = numRows * numCols;
const board = new Array(numEmptyCells);
const markers = ["x", "o"];
let player = 0;
let gameIsOver = false;
The variables above are declared in the script.js
(outside of any functions) and therefore have a global scope. It is generally not a good practice to declare globally scoped variables; we leave it at that for now and return to the topic in later chapters.
The script.js
also contains the function definition of toLinearIndex
:
// return the linear index corresponding to the row and column subscripts
function toLinearIndex(row, col) {
return row * numRows + col;
}
Notice numRows
is defined globally, and the function has access to it.
The game board is a 3 x 3 grid:
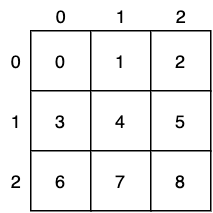
However, we store this board in a 1D array (board
):

The toLinearIndex
function, as the name suggests, returns the linear index into board
corresponding to the row and column subscripts of the game board.
The toLinearIndex
is a helper method. You will likely find a use for it when you implement the game. This function is defined in standard form using a function
keyword, followed by the function name, parameters, and the function body.
Note a JavaScript function can return a value (using the return statement). Unlike functions in Java and C++, functions in JavaScript have no parameter/return types.
Now, please implement the game!
My suggestion is to spend no more than 45 minutes on it. Then, check out the solution (next step).
As you implement the game, try to break it into smaller tasks and implement those tasks as functions. For example, you can have a function to switch players. As another example, you may want to have a function to check if all cells in a given row are marked with the same marker:
// returns true if all cells in the given row
// are marked with the same marker, otherwise returns false
function checkRow(row) {
return true;
}